Refactor blog into Node.js. Code color formatting
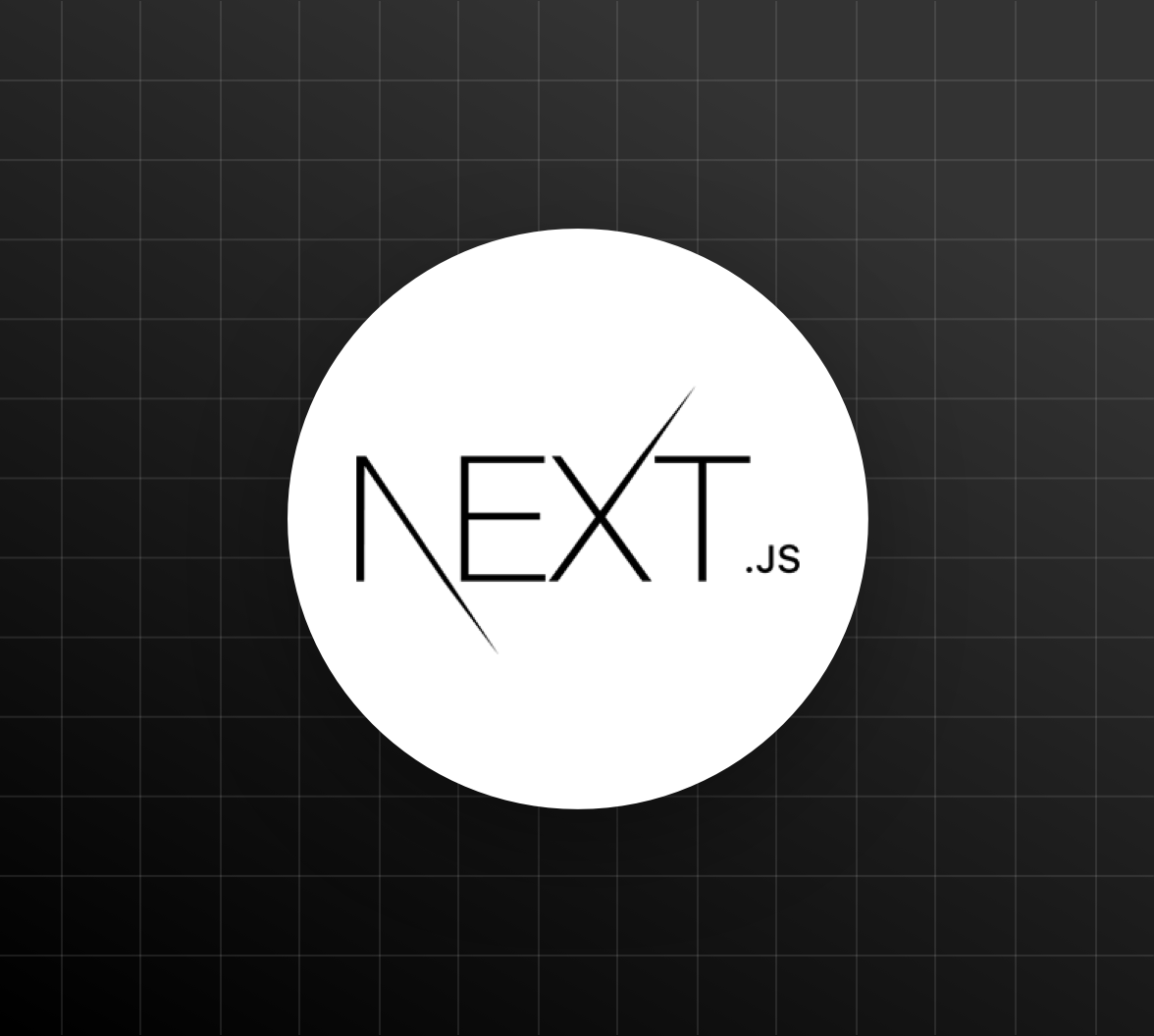
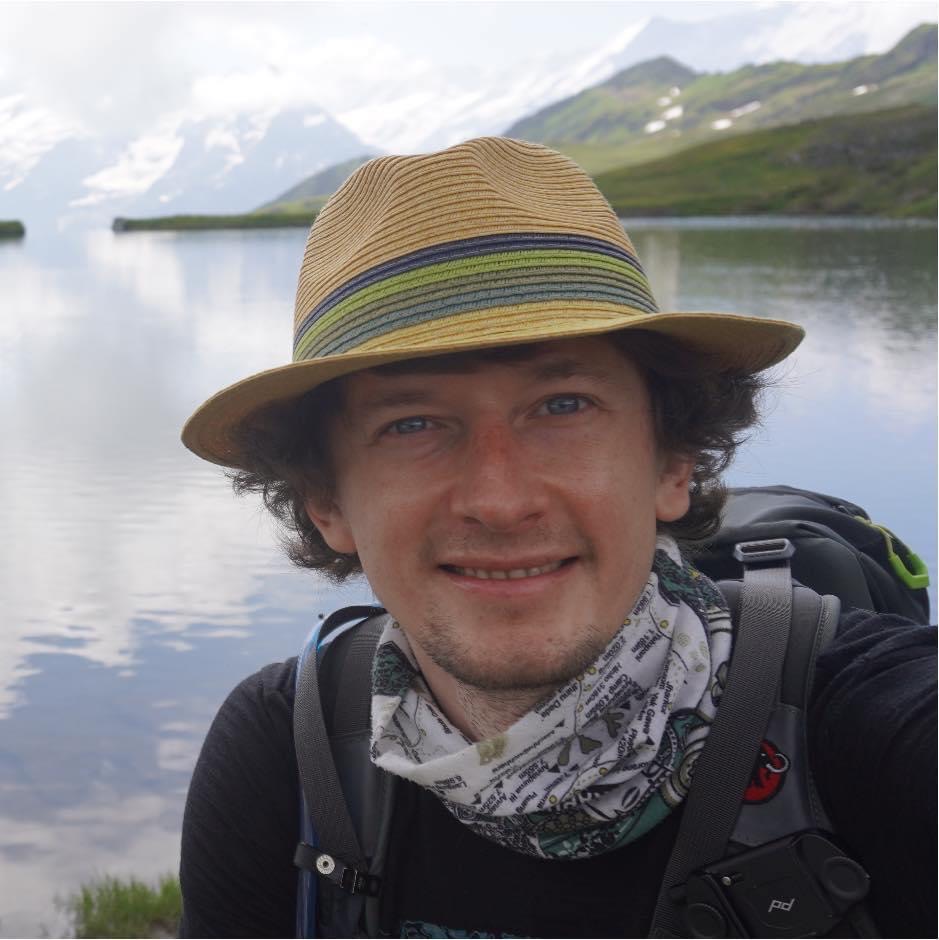
Sergej Brazdeikis
Ok I've seen already a plugin for this while reading the manual: https://github.com/remarkjs/react-markdown#use-custom-components-syntax-highlight
This example shows how you can overwrite the normal handling of an element by passing a component. In this case, we apply syntax highlighting with the seriously super amazing [
react-syntax-highlighter
][react-syntax-highlighter] by [@conorhastings][conor]:
import React from 'react' import ReactDom from 'react-dom' import ReactMarkdown from 'react-markdown' import {Prism as SyntaxHighlighter} from 'react-syntax-highlighter' import {dark} from 'react-syntax-highlighter/dist/esm/styles/prism' // Did you know you can use tildes instead of backticks for code in markdown? ✨ const markdown = `Here is some JavaScript code: ~~~js console.log('It works!') ~~~ ` ReactDom.render( <ReactMarkdown children={markdown} components={{ code({node, inline, className, children, ...props}) { const match = /language-(\w+)/.exec(className || '') return !inline && match ? ( <SyntaxHighlighter children={String(children).replace(/\n$/, '')} style={dark} language={match[1]} PreTag="div" {...props} /> ) : ( <code className={className} {...props}> {children} </code> ) } }} />, document.body )
Let's our favorite command first:
npm install react-syntax-highlighter
This needs small adjustments, and put it in our existing post-body.js
import ReactMarkdown from "react-markdown" import markdownStyles from "./markdown-styles.module.css" import { Prism as SyntaxHighlighter } from 'react-syntax-highlighter' const components = { code: code => { const match = /language-(\w+)/.exec(code.className || '') return !code.inline && match ? ( <SyntaxHighlighter children={String(code.children).replace(/\n$/, '')} language={match[1]} PreTag="div" /> ) : ( <code> {code.content} </code> ) } } export default function PostBody({ content }) { return ( <div className="max-w-2xl mx-auto"> <ReactMarkdown className={markdownStyles["markdown"]} children={content} components={components} /> </div> ) }
I removed dark theme, somehow didn't like it. To add it add in the top:
import {dark} from 'react-syntax-highlighter/dist/esm/styles/prism
and style={dark}
in the <SyntaxHighlighter>
component.
Cheers!